Overview
In this tutorial, we will learn how to detect eyes from an image using the Haar Cascades classifier. For the well-known tasks, the classifiers / detectors already exists, for example: detecting things like faces, cars, smiles, eyes and license plates.
Object Detection using Haar feature-based cascade classifiers is an effective object detection approach recommended by Paul Viola and Michael Jones in their paper, "Rapid Object Detection using a Boosted Cascade of Simple Features" in 2001.
Installing the modules
To install the OpenCV module and some other associated dependencies, we can use the pip command:
pip install opencv-python
pip install numpy
Download the Haar Cascades
- Follow the URL:
https://github.com/opencv/opencv/tree/master/data/haarcascades/ - Click on haarcascade_eye.xml
- Click on Raw and then press Ctrl + S. This will help you save the Haar Cascade file for eyes.
The Code
import cv2
import numpy as np
cascade = cv2.CascadeClassifier('haarcascade_eye.xml')
org_img = cv2.imread('kid.jpg') # reading image
img = cv2.resize(org_img, (500,500)) # resizing image size
copy = img.copy() # copying the image
gray = cv2.cvtColor(copy, cv2.COLOR_BGR2GRAY) # convert image
eyes = cascade.detectMultiScale(gray, 1.3, 5)
for (ex, ey, ew, eh) in eyes:
cv2.rectangle(copy, (ex, ey), (ex+ew, ey+eh), (155, 155, 0), 2)
stack = np.hstack([img, copy])
cv2.imshow('Output', stack)
cv2.waitKey(0)
The Ouput
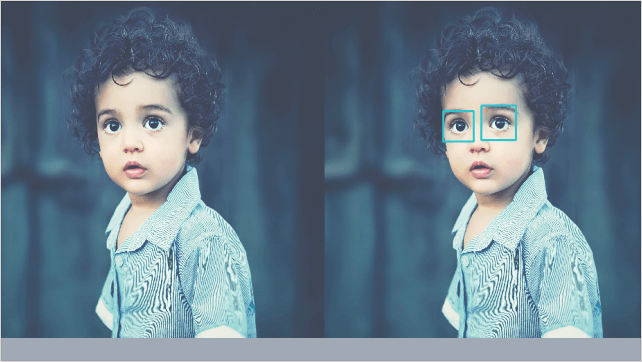